You can use this with all type of post types in wordpress. Like post, pages, products. You can add more custom fields in your woocommerce products.
Add a filter hook in your theme’s function.php file with ‘tp_meta_boxes’. Return a multidimensional array with your meta id, label/title, specify the post_type you want to add for the fields. Then add the fields inside the fields array.
- metabox_id // please give an id for distinguish them.
- title // for the label
- priority (core, 1,2,3…) // set to core
- context (normal, side) // Either you want the fields under the post or right side
- post_type (posts, pages or any custom posts type)
<?php
add_filter( 'tp_meta_boxes', 'themepure_metabox' ); function themepure_metabox( $meta_boxes ) { $meta_boxes[] = array( 'metabox_id' => '_tp_metabox', 'title' => esc_html__( 'Your Metabox Title', 'textdomain' ), 'post_type'=> 'post', // page, custom post type name 'context' => 'normal', 'priority' => 'core', 'fields' => array( array( 'label' => 'Text Field', 'id' => "_tp_text", 'type' => 'text', // specify the type field 'placeholder' => 'Type...', 'default' => '', 'conditional' => array() ), array( 'label' => 'Textarea Field', 'id' => "_tp_text", 'type' => 'textarea', // specify the type field 'placeholder' => 'Type...', 'default' => '', 'conditional' => array() ), array( 'label' => esc_html__( 'Image Field', 'textdomain' ), 'id' => "_tp_image", 'type' => 'image', // specify the type field 'default' => '', 'conditional' => array() ), array( 'label' => esc_html__( 'Gallery Field', '' ), 'id' => "_tp_gallery", 'type' => 'gallery', // specify the type field 'default' => '', 'conditional' => array() ), array( 'label' => esc_html__( 'Switch', 'textdomain' ), 'id' => "_tp_toggle", 'type' => 'switch', 'default' => 'off', 'conditional' => array() ), array( 'label' => esc_html__( 'Group Buttons Tab', '' ), 'id' => "_tp_buttons", 'desc' => '', 'type' => 'tabs', 'choices' => array( 'button_1' => 'Button 1', 'button_2' => 'Button 2', 'button_3' => 'Button 3', ), 'default' => 'button_1', 'conditional' => array() ), array( 'label' => esc_html__('Select Field', 'textdomain'), 'id' => "_tp_select", 'type' => 'select', 'options' => array( '1' => 'one', '2' => 'two ', '3' => 'three ', '4' => 'four ', '5' => 'five ', ), 'placeholder' => 'Select an item', 'conditional' => array(), 'default' => '' ), array( 'label' => 'Datepicker', 'id' => "_tp_date", 'type' => 'datepicker', 'placeholder' => '', 'default' => '', 'conditional' => array() ), array( 'label' => 'Colorpicker', 'id' => "_tp_color", 'type' => 'colorpicker', 'placeholder' => '', 'default' => '', 'conditional' => array() ), array( 'label' => esc_html__('Posts Select Field', 'textdomain'), 'id' => "_tp_posts", 'type' => 'select_posts', 'post_type' => 'post', // specify the post type you want to fetch 'placeholder' => 'Select a post', 'conditional' => array(), 'default' => '' ), array( 'label' => esc_html__('Repeater Field', 'textdomain'), 'id' => "_tp_repeater", 'type' => 'repeater', // specify the type "repeater" (case sensitive) 'conditional' => array(), 'default' => array(), 'fields' => array( array( 'label' => esc_html__('Your Title', 'textdomain'), 'id' => "_tp_r_select", 'type' => 'select', 'options' => array( 'footer_1' => 'Footer 1', 'footer_2' => 'Footer 2', 'footer_3' => 'Footer 3' ), 'placeholder' => 'Select a footer', 'conditional' => array(), 'default' => 'footer_1', ), array( 'label' => esc_html__('Select Footer Style', 'textdomain'), 'id' => "_footer_style_2", 'type' => 'select', 'options' => array( 'footer_1' => 'Footer 1', 'footer_2' => 'Footer 2', 'footer_3' => 'Footer 3' ), 'placeholder' => 'Select a footer', 'conditional' => array(), 'default' => 'footer_1', 'bind' => "_footer_template_2" // bind the key to be control with conditions ), array( 'label' => esc_html__('Select Footer Template', 'textdomain'), 'id' => "_footer_template_2", 'type' => 'select_posts', 'placeholder' => 'Select a template', 'post_type' => 'tp-footer', 'conditional' => array( "_footer_style_2", "==", "footer_2" // First parameter will be the id of control field and so on ), 'default' => '', ) ) ) ), ); return $meta_boxes; }
?>
Conditional Field #
You can specify a conditional field with the key conditional. Just give 3 parameters inside the conditional array.
- 1st paremeter is the id of any fieldÂ
- 2nd parameter is the operator (==, <=, >=, <, >, !=) to comapre the value.
- 3rd parametr is for the value you want to compare with.
<?php
array(
'label' => 'Text Field',
'id' => "_your_id",
'type' => 'text',
'placeholder' => '',
'default' => '',
'conditional' => array(
"_id_of_any_field", "any operator", "value_of_that_field"
)
)
array(
'label' => 'Text Field',
'id' => "_your_id",
'type' => 'text',
'placeholder' => '',
'default' => '',
'conditional' => array(
"_field_id", "==", "_field_value"
)
)
?>
For Binding With The Post Format #
Specify a key called post_format inside the parent array. The default wordpress post formats are. (gallery, audio, video, aside). Se the example below:
<?php
add_filter( 'tp_meta_boxes', 'themepure_metabox' );
function themepure_metabox( $meta_boxes ) {
$meta_boxes[] = array(
'metabox_id' => $prefix . '_post_meta_gallery_box',
'title' => esc_html__( 'Post Meta Gallery', 'donafund' ),
'post_type'=> 'post',
'context' => 'normal',
'priority' => 'core',
'fields' => array(
array(
'label' => esc_html__( 'Gallery Format', 'textdomain' ),
'id' => "{$prefix}_gallery_5",
'type' => 'gallery',
'default' => '',
'conditional' => array(),
),
),
'post_format' => 'gallery' // if u want to bind with post formats
);
$meta_boxes[] = array(
'metabox_id' => $prefix . '_post_meta_audio_box',
'title' => esc_html__( 'Post Meta Audio', 'donafund' ),
'post_type'=> 'post',
'context' => 'normal',
'priority' => 'core',
'fields' => array(
array(
'label' => esc_html__( 'Audio Format', 'donafund' ),
'id' => "{$prefix}_audio_format",
'type' => 'text',
'placeholder' => esc_html__( 'Audio url here', 'donafund' ),
'default' => '',
'conditional' => array()
),
),
'post_format' => 'audio' // if u want to bind with post formats
);
$meta_boxes[] = array(
'metabox_id' => $prefix . '_post_meta_video_box',
'title' => esc_html__( 'Post Meta Video', 'donafund' ),
'post_type'=> 'post',
'context' => 'normal',
'priority' => 'core',
'fields' => array(
array(
'label' => esc_html__( 'Video Format', 'donafund' ),
'id' => "{$prefix}_video_format",
'type' => 'text',
'placeholder' => esc_html__( 'Video url here', 'donafund' ),
'default' => '',
'conditional' => array()
),
),
'post_format' => 'video' // if u want to bind with post formats
);
return $meta_boxes;
}
?>
Specify columns for the view #
Specify a key called columns inside the parent array. Give a number liek 1,2,3….It will determine as columns value and It will view in admin dashboard accordingly. Se the example below:
<?php
add_filter( 'tp_meta_boxes', 'themepure_metabox' );
function themepure_metabox( $meta_boxes ) {
$meta_boxes[] = array(
'metabox_id' => $prefix . '_post_meta_gallery_box',
'title' => esc_html__( 'Post Meta Gallery', 'donafund' ),
'post_type'=> 'post',
'columns' => 2 // specify the value as numeric number
'context' => 'normal',
'priority' => 'core',
'fields' => array(
array(
'label' => esc_html__( 'Gallery Format', 'textdomain' ),
'id' => "{$prefix}_gallery_5",
'type' => 'gallery',
'default' => '',
'conditional' => array(),
),
),
);
return $meta_boxes;
}
?>
2 Columns #
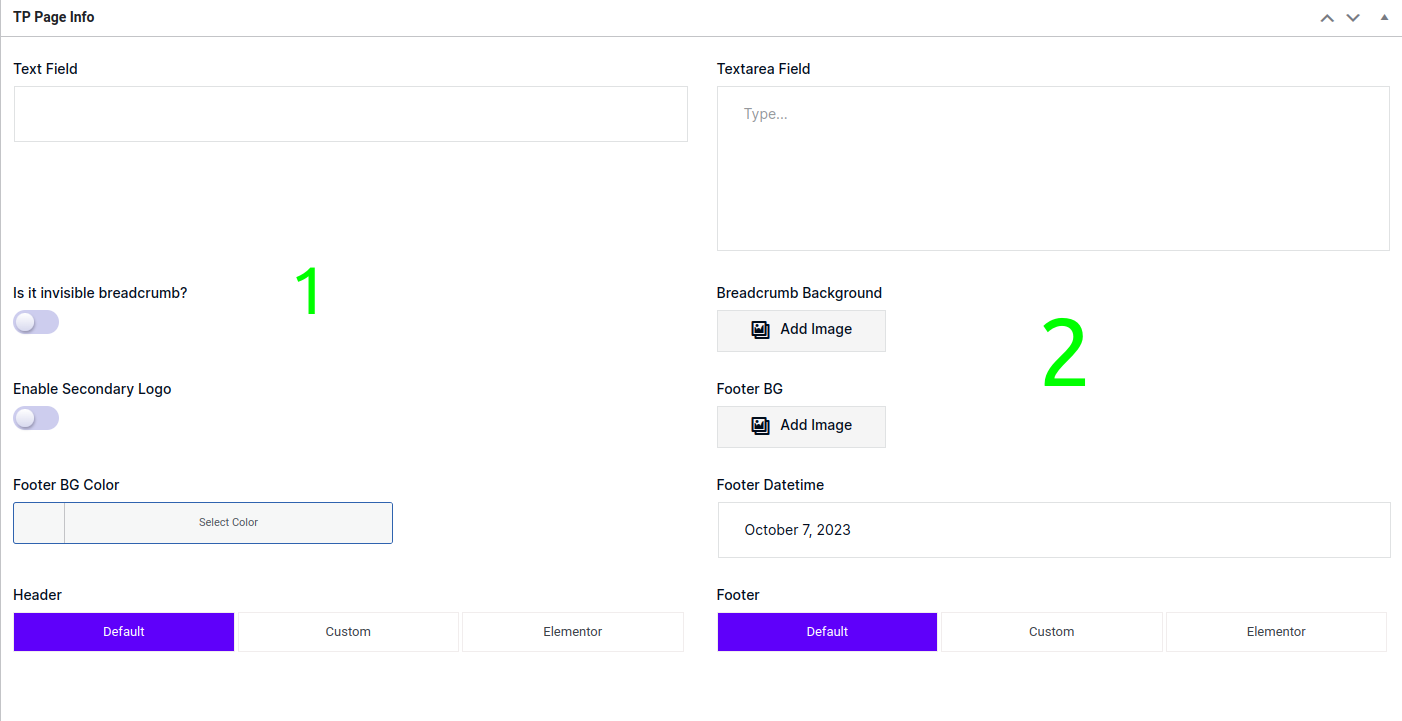
<?php
add_filter( 'tp_meta_boxes', 'themepure_metabox' );
function themepure_metabox( $meta_boxes ) {
$meta_boxes[] = array(
'metabox_id' => $prefix . '_post_meta_gallery_box',
'title' => esc_html__( 'Post Meta Gallery', 'donafund' ),
'post_type'=> 'post',
'columns' => 5 // specify the value as numeric number
'context' => 'normal',
'priority' => 'core',
'fields' => array(
array(
'label' => esc_html__( 'Gallery Format', 'textdomain' ),
'id' => "{$prefix}_gallery_5",
'type' => 'gallery',
'default' => '',
'conditional' => array(),
),
),
);
return $meta_boxes;
}
?>
5 Columns #
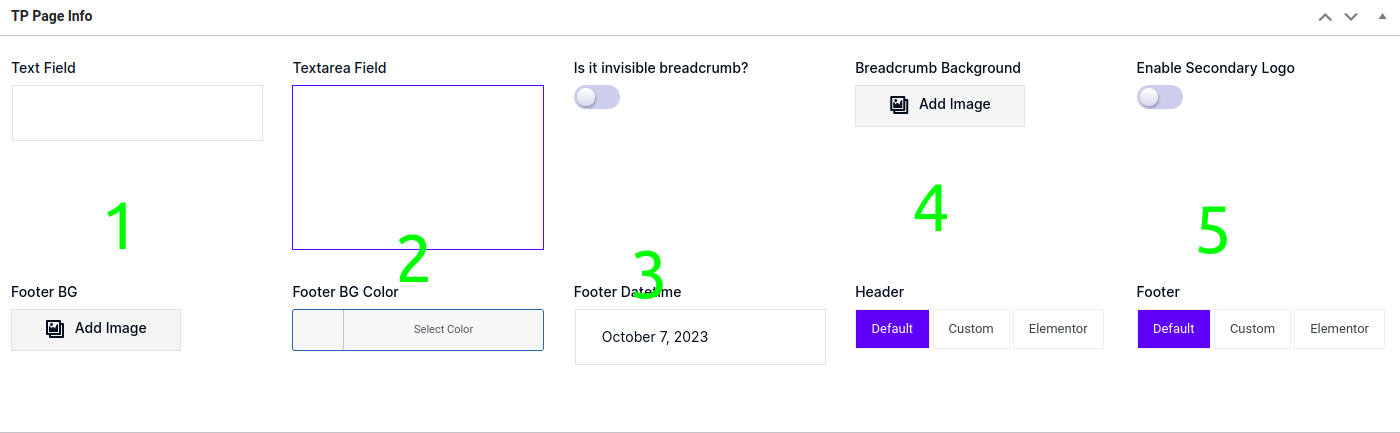